58
views
views
This provides a solid foundation for creating an interactive wall clock using JavaScript and HTML. Feel free to customize and expand upon these concepts to create a unique and engaging clock experience.
-
HTML Structure:
- Create an HTML file for create wall clock using javascript (e.g.,
index.html
). - Add an empty
<div>
element with an ID to act as the clock's container (e.g.,clock-container
). - Include a
<script>
tag to link your JavaScript file (e.g.,script.js
).
- Create an HTML file for create wall clock using javascript (e.g.,
-
CSS Styling (Optional):
- Create a CSS file (e.g.,
style.css
) or style directly within the<style>
tag in your HTML. - Style the clock container and its elements (hands, numbers, etc.) for visual appeal.
- Create a CSS file (e.g.,
2. JavaScript Implementation
-
Get Clock Elements:
- Use JavaScript's
document.getElementById()
to access the clock container and any other relevant elements.
- Use JavaScript's
-
Function to Get Current Time:
- Create a function (e.g.,
getCurrentTime()
) that:- Gets the current date and time using
new Date()
. - Extracts hours, minutes, and seconds from the date object.
- Calculates the angle for each hand (hours, minutes, seconds) based on their respective values.
- Hour Hand:
- Calculate the angle by dividing the hours by 12 and multiplying by 360 degrees.
- Add the minutes/60 to account for the hour hand's movement within the hour.
- Minute Hand:
- Calculate the angle by dividing the minutes by 60 and multiplying by 360 degrees.
- Second Hand:
- Calculate the angle by dividing the seconds by 60 and multiplying by 360 degrees.
- Hour Hand:
- Gets the current date and time using
- Create a function (e.g.,
-
Function to Rotate Hands:
- Create a function (e.g.,
rotateHands()
) that:- Calls
getCurrentTime()
to get the current time and angles. - Uses CSS
transform: rotate()
to dynamically rotate each hand element based on the calculated angles.
- Calls
- Create a function (e.g.,
-
Update Clock Regularly:
- Use
setInterval()
to repeatedly call therotateHands()
function at a specific interval (e.g., every 1 second). This ensures the clock updates in real-time.
- Use
3. Example Code Snippet (JavaScript)
JavaScript
function getCurrentTime() {
const now = new Date();
const hours = now.getHours() % 12 || 12; // Handle 24-hour format
const minutes = now.getMinutes();
const seconds = now.getSeconds();
// Calculate angles for each hand
const hourAngle = (hours + minutes / 60) * (360 / 12);
const minuteAngle = (minutes + seconds / 60) * (360 / 60);
const secondAngle = seconds * (360 / 60);
return { hours, minutes, seconds, hourAngle, minuteAngle, secondAngle };
}
function rotateHands() {
const { hourAngle, minuteAngle, secondAngle } = getCurrentTime();
// Get hand elements (assuming you have elements with IDs)
const hourHand = document.getElementById('hour-hand');
const minuteHand = document.getElementById('minute-hand');
const secondHand = document.getElementById('second-hand');
// Rotate hands using CSS transform
hourHand.style.transform = `rotate(${hourAngle}deg)`;
minuteHand.style.transform = `rotate(${minuteAngle}deg)`;
secondHand.style.transform = `rotate(${secondAngle}deg)`;
}
// Update clock every second
setInterval(rotateHands, 1000);
4. Enhancements (Optional)
- Add Clock Face:
- Create a circular clock face with numbers or markers.
- Use Canvas:
- For more advanced customization, use the HTML Canvas API to draw the clock elements directly on the canvas.
- Add Styling:
- Apply CSS styles to make the clock visually appealing (colors, fonts, shadows, etc.).
- Add Analog/Digital Functionality:
- Include an option to switch between analog and digital clock displays.
Key Considerations:
- Browser Compatibility:
- Test your clock in different browsers to ensure cross-browser compatibility.
- Performance:
- Optimize your code for smooth and efficient clock updates.
This provides a solid foundation for creating an interactive wall clock using JavaScript and HTML. Feel free to customize and expand upon these concepts to create a unique and engaging clock experience.
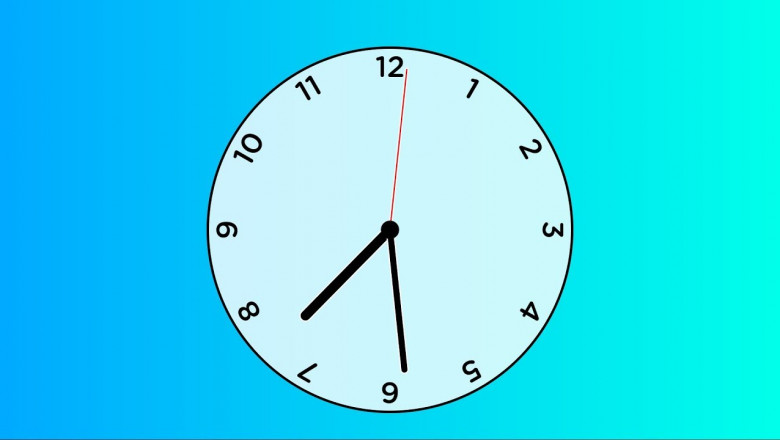

Comments
0 comment