views
PHP Email Validation: Best Practices for Clean and Reliable User Input
Introduction
Email validation is one of the most fundamental yet crucial tasks in any web application. Whether you’re building a contact form, signup page, or newsletter system, collecting valid email addresses ensures smooth user communication, reduces bounce rates, and helps maintain a healthy database.
In the world of PHP email validation goes beyond just checking if an email looks correct—it’s about securing your site from bad data and potential exploits. In this guide, we’ll walk you through PHP Email Validation Best Practices for Clean and Reliable User Input, covering everything from basic syntax checks to advanced sanitization techniques.
Let’s dive in.
Why Email Validation Matters
Before we jump into the code, let’s quickly understand why validating email addresses is important:
-
Data Quality: Avoids storing fake or malformed email addresses.
-
Security: Prevents malicious input that could be used in email injection attacks.
-
Functionality: Ensures system emails (e.g., password resets or newsletters) are sent to real users.
-
User Experience: Helps users correct typos in real-time.
Skipping proper validation can cause issues down the road, from broken email workflows to security vulnerabilities. That’s why it’s a best practice to validate every user input—especially emails.
Built-In Email Validation in PHP
The easiest and most common way to validate email addresses in PHP is by using the built-in filter_var()
function.
How It Works
-
FILTER_VALIDATE_EMAIL
checks the syntax of the email address using RFC standards. -
It returns the email if it’s valid or
false
if it’s not.
While filter_var()
is simple and effective for basic validation, it should be part of a more comprehensive email validation process.
Sanitizing Email Inputs
Before you validate, it’s essential to sanitize. Sanitization removes unwanted or potentially harmful characters from input.
Use FILTER_SANITIZE_EMAIL
to clean the email string:
This strips out illegal characters like spaces, control characters, and even scripts that could cause security issues.
Best Practice: Always sanitize before validating. This ensures the input is clean before running checks on it.
Regex vs. Built-in Validation
Some developers prefer using regular expressions (regex) for email validation, especially when they want to enforce stricter rules.
Here’s an example:
While regex can give you control, it comes with drawbacks:
-
Harder to maintain and debug
-
Might miss valid emails or allow invalid ones
-
Doesn’t follow the full RFC 5322 spec
Recommendation: Stick with PHP’s built-in filters unless you have a specific reason to use regex.
Validating Email Domains (MX Record Check)
What if an email is syntactically valid but the domain doesn’t exist? That’s where checking the domain's MX (Mail Exchange) records helps.
Why It’s Useful
-
Ensures the domain can receive emails
-
Helps prevent spam and fake registrations
This step is optional but highly recommended for systems dealing with high email traffic or requiring secure signups.
Real-Time Email Validation (Client-Side + Server-Side)
Although PHP handles server-side validation, pairing it with JavaScript for real-time validation improves the user experience.
Example using HTML5:
JavaScript validation:
Combine Both
Even with front-end validation, always validate again on the server. Never trust client input completely—users can bypass scripts or manipulate forms.
Protecting Against Email Injection
Email injection is an attack where malicious users inject headers or scripts into form fields to send spam through your server.
Vulnerable Example:
If a user submits an email like:
…it could send unwanted emails.
Solution: Strict Validation + Header Injection Check
Best Practices Recap
Here’s a summary of best practices to follow when handling PHP email validation:
-
✅ Sanitize first using
FILTER_SANITIZE_EMAIL
-
✅ Validate using
FILTER_VALIDATE_EMAIL
-
✅ Check MX records for domain validity (if necessary)
-
✅ Avoid using complex regex unless needed
-
✅ Combine server-side and client-side validation
-
✅ Prevent email injection by filtering control characters
-
✅ Log failed attempts for security audits or debugging
Use Case: Signup Form Example
Let’s tie it all together in a simple signup form:
This sample ensures the input is clean, secure, and comes from a valid email domain.
Final Thoughts
Validating email addresses may seem like a small step, but it plays a huge role in securing your application and ensuring quality data. With spam, bots, and user errors lurking around every form field, taking the time to implement validation properly will pay off in better performance and fewer headaches.
By following the principles in this guide on PHP Email Validation: Best Practices for Clean and Reliable User Input, you’re setting your app up for clean data, solid user experience, and peace of mind.
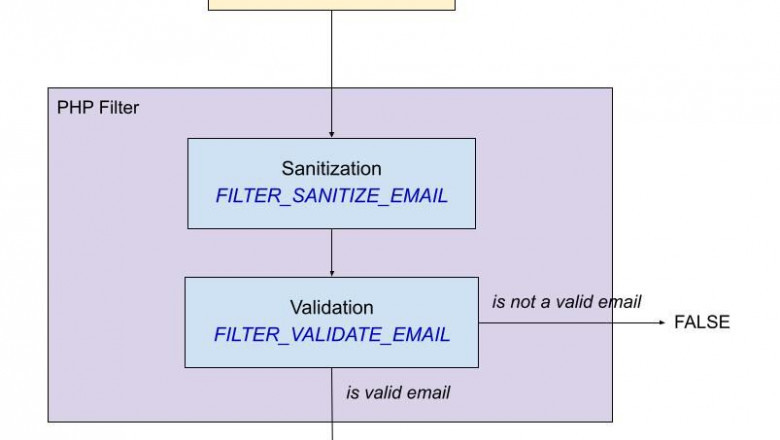

Comments
0 comment