views
Demystifying Java Memory Management: How Garbage Collection Really Works
Demystifying Java Memory Management: How Garbage Collection Really Works
"Memory, like time, slips quietly away when left unmanaged."
– A developer on the brink ofOutOfMemoryError
Java, for all its verbosity and structure, does one thing gracefully: memory management. And at the heart of that is Garbage Collection (GC)—a process often discussed, seldom understood. To many, it feels like magic. But in reality, it’s engineering, not illusion.
Let’s slow down, peel back the layers, and understand how Java keeps your memory in check—even when your code doesn’t.
The Java Memory Model: The Silent City
Imagine the JVM as a city. In this city, memory is divided into regions:
-
Heap – The main town where objects live.
-
Stack – The swift alleys where method calls and local variables come and go.
-
Method Area – The blueprint district for class metadata and static data.
-
Program Counter Register – The navigator.
-
Native Method Stack – Where non-Java stories are told.
The Heap, in particular, is where garbage collection does its rounds.
The Lifecycle of an Object
When you use new
, an object is born. It lives in the Young Generation—a nursery. If it survives a few rounds of cleaning, it graduates to the Old Generation, where long-living objects stay. But not all objects get to grow old.
Garbage Collection steps in when memory begins to fill. It sweeps, sorts, and clears what’s no longer reachable.
Who Decides What’s Garbage?
Simple rule: If nothing in your code can reach an object, it’s garbage.
Java uses something called reachability analysis, tracing object references from “GC roots”—like active threads, static fields, and method parameters. If no path connects to an object, it's lost. Forgotten. Reclaimed.
Types of Garbage Collectors in Java (2025 Edition)
-
Serial GC – Simple, single-threaded, good for small apps.
-
Parallel GC – Multi-threaded, default for most.
-
CMS (Deprecated) – Was low-latency, now retired.
-
G1 GC – Focuses on predictable pause times, a balance of speed and memory.
-
ZGC / Shenandoah – Ultra-low pause collectors, ideal for massive heaps.
Each GC type carries its rhythm. Understanding which one your JVM is dancing with can make all the difference.
Pause. Clean. Resume.
Garbage collection isn’t always invisible. Your app pauses when GC runs—sometimes subtly, sometimes painfully. Tuning GC behavior is often about managing these pauses.
Tools like VisualVM, JConsole, or modern profilers help observe these cycles. Logs can show how often GC is triggered, how much memory it reclaims, and whether your app is struggling with frequent cleanups.
Myths That Deserve a Quiet Burial
-
“GC means I don’t need to worry about memory leaks.”
Not true. Holding references unnecessarily can prevent collection. -
“More memory means faster performance.”
Sometimes, a bigger heap just delays GC and causes longer pauses when it happens. -
“Finalizers are reliable.”
No. Use them only when absolutely necessary. Prefertry-with-resources
.
Why It Matters to You
If you're building Java applications—whether backend systems, Android apps, or enterprise software—understanding how memory is managed isn’t optional. It's part of the craftsmanship. Your users don’t see it. But they feel it—when an app runs smooth, when it doesn't crash, when it's quiet even under pressure.
The best Java developers aren’t just writers of syntax. They are quiet choreographers of memory.
https://kphb.nareshit.com/core-java-training-in-kphb/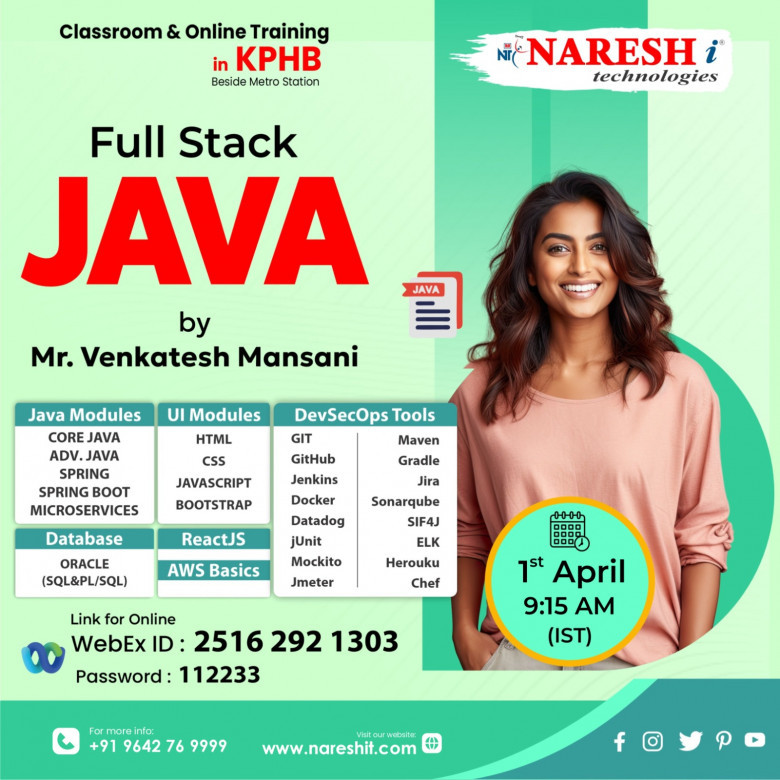
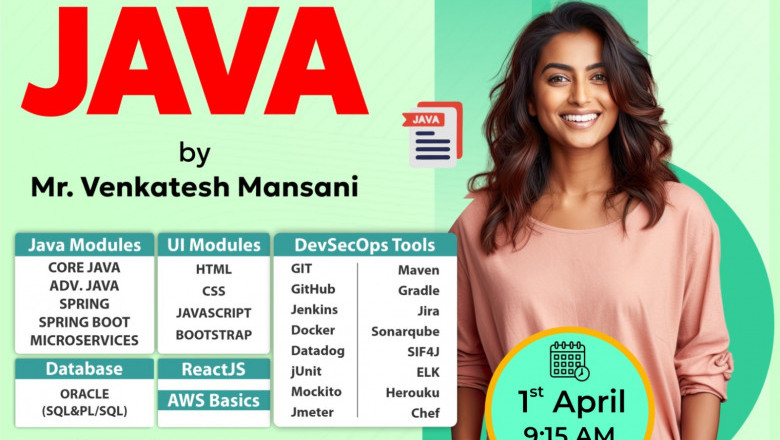
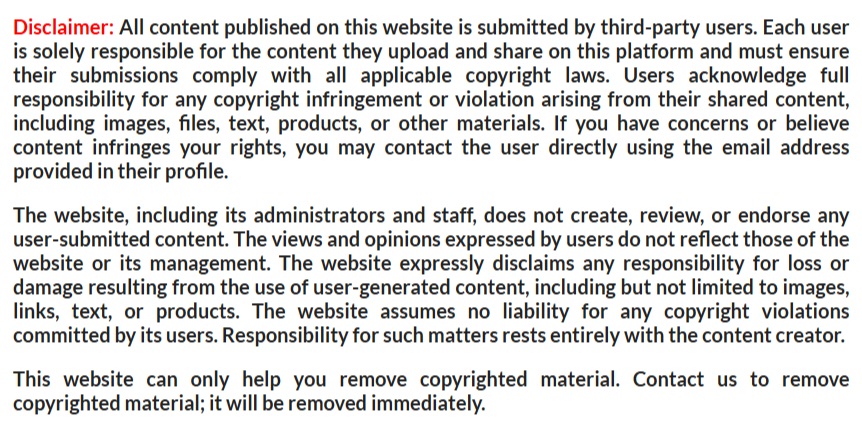
Comments
0 comment